For this assignment I was tasked with creating a device using an Arduino, and from that I was basically given free rein to make whatever I wanted. I decided to try to recreate as best I could a traffic light. Before I creating this though, I created a flowchart to map out my process and have stages that I could clearly follow.
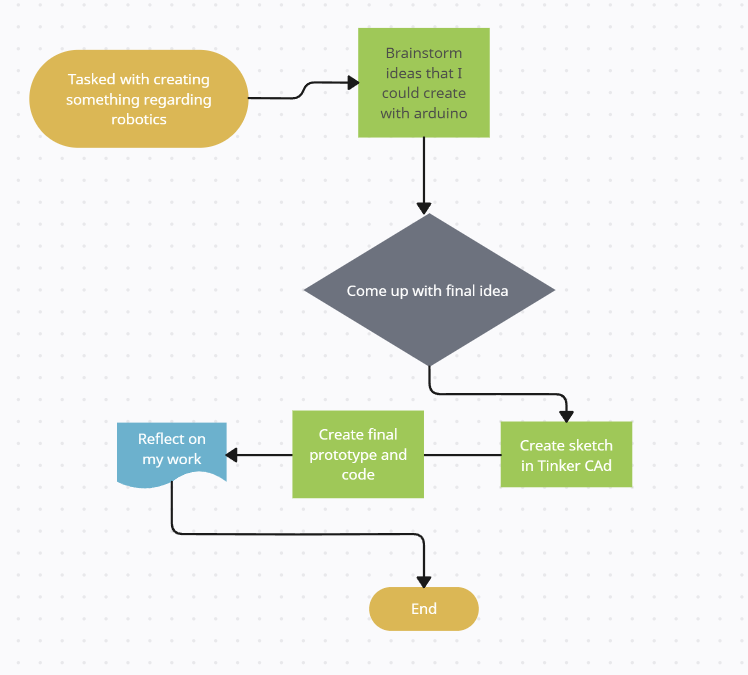
Firstly, I decided to create a sketch of what this project would look like in tinker Cad, as well as create the basic code that would go with my project:
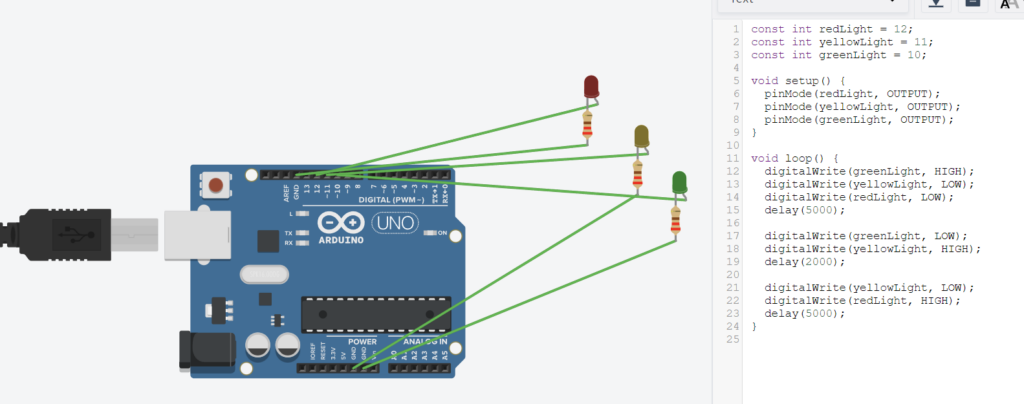
Next, I then started making the actual circuit, and made a design change to use a breadboard to help organize my wires instead of plugging directly. Once I had created the initial circuit, I then decided that I also wanted to add a button that when pressed, would make the red light time go from 5 seconds to 2.5 seconds, to simulate when someone presses the crosswalk button.
Here is the code if you would like to create this for yourself:
const int redLight = 12;
const int yellowLight = 11;
const int greenLight = 10;
const int buttonPin = 2;
const unsigned long defaultRedLightTime = 5000;
const unsigned long yellowLightTime = 2000;
const unsigned long greenLightTime = 5000;
// Create a variable to store the actual red light time for each cycle
unsigned long redLightTime = defaultRedLightTime;
void setup() {
// Set up the LED pins
pinMode(redLight, OUTPUT);
pinMode(yellowLight, OUTPUT);
pinMode(greenLight, OUTPUT);
// Set button pin as input with internal pull-up resistor
pinMode(buttonPin, INPUT_PULLUP);
Serial.begin(9600);
}
void loop() {
digitalWrite(greenLight, HIGH);
digitalWrite(yellowLight, LOW);
digitalWrite(redLight, LOW);
delay(greenLightTime);
// *** YELLOW LIGHT PHASE ***
digitalWrite(greenLight, LOW);
digitalWrite(yellowLight, HIGH);
digitalWrite(redLight, LOW);
delay(yellowLightTime);
// Check if button is pressed when entering the red light phase
int buttonState = digitalRead(buttonPin);
if (buttonState == LOW) {
redLightTime = defaultRedLightTime / 2;
Serial.println(“Button pressed: Red light time halved to 2.5 seconds”);
} else {
redLightTime = defaultRedLightTime;
Serial.println(“Button not pressed: Red light time set to 5 seconds”);
}
digitalWrite(greenLight, LOW);
digitalWrite(yellowLight, LOW);
digitalWrite(redLight, HIGH);
delay(redLightTime);
}
Additionally, if you would like to create this circuit you will need:
One Arduino
One Breadboard
3 Resistors
3 LED’s ranging in color
One button
Approximately 10 wires for connecting
Leave a Reply