For this assignment, I was tasked with using my coding knowledge to create some sort of code that can help solve a real world problem. I thought about what I could create with my fairly limited knowledge of coding, and decided to create a currency calculator, and a quiz on how to manage your money. I decided to create this, as a lot of kids grow up not knowing how to manage their money, and as a result end up in debt and other sorts of financial trouble. The programming language I am using is Python, which is the most common coding language and is used in majority of the apps we use today. Some of these apps include Instagram, Google, and Youtube. The currency calculator works like this. You take the base currency you want to use and input it into the calculator. You would then search up the conversion rate between the two currencies and input it into the calculator. The calculator would then calculate the amount of money in the currency you want. Let’s do an example together. For this example, lets convert $500 (CAD) and convert it into USD. I would input 500 into the calculator, and then the conversion rate, which is 0.74. The calculator would then calculate the amount of money in USD which is $370. This Calculator is very helpful if you are going on vacation and need to figure out how much money you need to bring. If I could improve this further, I would add built in currencies so you wouldn’t even need to input the conversion rate and the calculator could do it on its own. I created this piece of code using the bases of this flow chart.
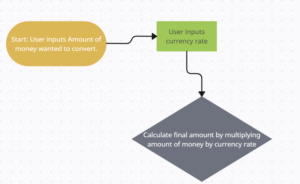
Here is the code if you would like to input it into a console and try it for yourself:
# Currency Calculator
def currency_converter(amount, exchange_rate):
“””
Converts one currency to another based on the given exchange rate.
Parameters:
amount (float): The amount of money to convert.
exchange_rate (float): The conversion rate from one currency to another.
Returns:
float: The converted currency value.
“””
return amount * exchange_rate
# Example Usage:
amount = float(input(“Enter the amount to convert: “))
exchange_rate = float(input(“Enter the exchange rate: “))
converted_amount = currency_converter(amount, exchange_rate)
print(f”The converted amount is: {converted_amount:.2f}”)
Next, on the topic of money and currency, I decided to create a small quiz to help people who are uneducated in saving money. The quiz has 3 questions, and based on the score you get it will display a message based on your score. If you get only one or no questions right, it will display “You need more practice. Keep learning about finances!” If you get 2 questions right it will display, “Good job! You passed the quiz.” If you get all the questions correct, it will display “Great! You have solid financial knowledge.” I once again created this piece of code based off of this flow chart:
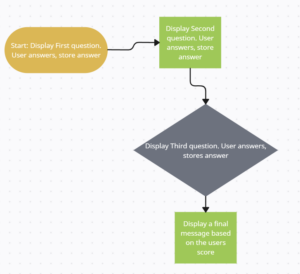
If you would like to try the quiz, here is the code that you can copy into a console:
import random #Import the randomize function so the questions display in a different order
def finance_quiz():
questions = [
(“What is a good strategy for saving money?”,
[“a) Create a budget”, “b) Spend without tracking”, “c) Avoid saving”], “a”), #
(“What does ‘APR’ stand for in financial terms?”,
[“a) Annual Percentage Rate”, “b) Average Price Rating”, “c) Additional Payment Required”], “a”),
(“What is considered a good credit score?”,
[“a) 700 or above”, “b) 500-600”, “c) Below 400”], “a”)
]
random.shuffle(questions)
total_questions = 0
correct_answers = 0
print(“Financial Literacy Quiz”)
for question, choices, correct_answer in questions: #Setting up the code to count the score
total_questions += 1
print(question)
for choice in choices:
print(choice)
answer = input(“> “).lower().strip() # Ensure input is lowercased and stripped of spaces
if answer == correct_answer:
print(“Correct!”)
correct_answers += 1
else:
correct_choice = [choice for choice in choices if choice.startswith(correct_answer)][0]
print(f”Incorrect. The correct answer is {correct_choice}”)
print(“\nQuiz complete!”)
print(f”You answered {correct_answers} out of {total_questions} questions correctly.”) #Displaying final score
if correct_answers == total_questions: #Displaying message based on your score
print(“Great! You have solid financial knowledge.”)
elif correct_answers >= total_questions / 2:
print(“Good job! You passed the quiz.”)
else:
print(“You need more practice. Keep learning about finances!”)
# Example Usage
finance_quiz()
Here is a free online console. Simply copy the code in and hit run.
Leave a Reply