Hello all! Have you ever wanted to create musical tunes on the fly? If you have, then look no further. This is my first Arduino project for the year and it is a simple Arduino Piano.
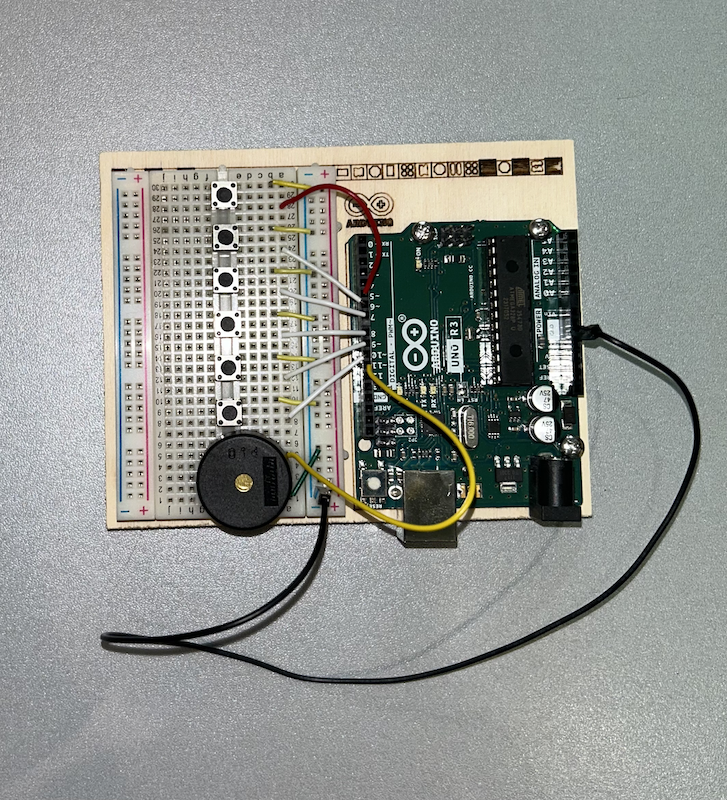
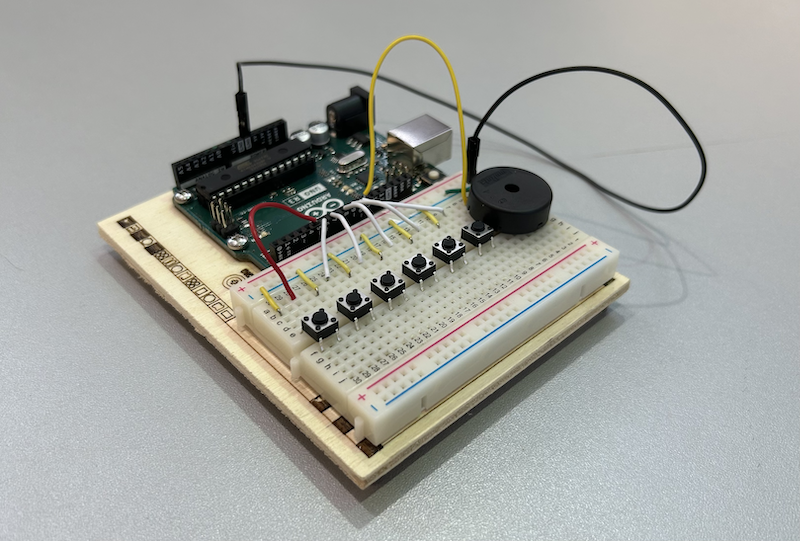
Components and Supplies
- Breadboard
- Jumper Wires
- Arduino UNO
- Buzzer (I use the Murata PKM22EPP-40)
- Buttons
Wiring
The wiring for this contraption is very simple. The buttons are connected to D5 to D10 and the piezo buzzer goes into D11 of the Arduino. They also all go into GND.
Coding
The coding for the piano is unfortunately a little more complicated than the wiring, although still relatively simple.
I first defined the musical note frequencies as constants. These frequencies represent different pitches in Hertz. You can use any notes you want, but I have used the notes C – A from the fourth octave in this code.
Then, I created two arrays and a buzz
variable to make the process easier:
notes[]
, which contain the pin numbers for the input buttons (10, 9, 8, 7, 6, 5).tones[]
, which contain the corresponding frequencies (C, D, E, F, G, A) that are played when the corresponding button is pressed.buzz
contains the pin number for the buzzer that plays the tones (11).
In the setup()
function, I used a loop to iterate through the notes[]
array to initialize each button as an input with an internal pull-up resistor using pinmode()
. The pull-up resistor ensures that the input pin reads HIGH(no button pressed) by default and reads LOW
when the button is pressed.
The loop()
function continuously checks the state of each button using multiple if statements. The program checks each button in sequence using digitalRead(). If a button is pressed(LOW), the corresponding note is played using the tone() function, which sends the frequency to the buzzer connected to pin 11. If no button is pressed, noTone(buzz)
is called to stop the sound.
#define C 262
#define D 294
#define E 330
#define F 349
#define G 392
#define A 440
const int notes[] = {10, 9, 8, 7, 6, 5};
const int tones[] = {C, D, E, F, G, A};
const int buzz = 11;
void setup() {
for (int i = 0; i < 6; i++) {
pinMode(notes[i], INPUT);
digitalWrite(notes[i], HIGH);
}
}
void loop() {
while(1){
if(digitalRead(notes[0]) == LOW){
tone(buzz, tones[0]);
} else if (digitalRead(notes[1]) == LOW){
tone(buzz, tones[1]);
} else if (digitalRead(notes[2]) == LOW){
tone(buzz, tones[2]);
} else if (digitalRead(notes[3]) == LOW){
tone(buzz, tones[3]);
} else if (digitalRead(notes[4]) == LOW){
tone(buzz, tones[4]);
} else if (digitalRead(notes[5]) == LOW){
tone(buzz, tones[5]);
} else {
noTone(buzz);
}
}
}
Here is how it should look and work at the end:
Thank you for reading this blog post. I hope you have a nice day!
Leave a Reply