Hi, this is Aiden again and this is my Coding Assignment for my Fusion 10 program.
This is the link to my Python program. The code’s a little too long to show, but it’s a comedy machine that can generate jokes based on a subject and object input. You can choose to generate 1-10 jokes. You also get the option to choose an adjective if you select 10 jokes. The machine can regenerate jokes as many times as you want.
So basically how the COMEDY MACHINE works is: it goes through the main stuff, print(“comedy machine v1.0”), etc. Then it calls the function switch(), which is just a y/n verification to the next part.
After that, if your input is “y”, it’ll call the function which everything is based on: firststage(). (it just quits if you type “n”)
# firststage() houses all the jokes and jokesets for generation if humorlvl is set to 1.
def firststage():
global jokesub1, jokeobj1, jokeadj1
# this defines jokeset so that it can be converted to an int.
jokeset = jokeset_func()
try:
jokeset_int = int(jokeset)
except ValueError:
jokeset_int = None
# this is the error message for if the user doesn't input a number or a valid code.
if jokeset_int is None:
print("invalid code")
firststage()
# this is the error message for if the user inputs a number that is not between 1 and 10.
if jokeset_int is not None and jokeset_int < 0 or jokeset_int > 10:
print("please input a number from 1-10.")
firststage()
# this is the generation for if jokeset is from 1-9.
elif jokeset_int is not None and jokeset_int >= 1 and jokeset_int < 10:
print("great")
time.sleep(0.5)
jokesub1 = str(input("enter a subject: "))
jokeobj1 = str(input("enter an object: "))
print("great")
time.sleep(0.5)
verification01 = str(input("would you like to generate the jokes? (y/n) "))
if verification01 == "n":
firststage()
if verification01 == "y":
for i in range(jokeset_int):
joke = jokemaker()
print(joke)
time.sleep(0.2)
verification001 = str(input("would you like to regenerate the jokes?: (y/n) "))
if verification001 == "y":
refirststage()
if verification001 == "n":
print("thank you for using the comedy machine.")
time.sleep(1)
os.system("cls")
print("the comedy machine will always return.")
time.sleep(1)
exit()
else:
print("please choose between y or n.")
# this is the generation for if jokeset is 10.
elif jokeset_int is not None and jokeset_int == 10:
print("great")
time.sleep(0.5)
jokesub1 = str(input("enter a subject: "))
jokeobj1 = str(input("enter an object: "))
jokeadj1 = str(input("enter an adjective: "))
print("great")
time.sleep(0.5)
verification01 = str(input("would you like to generate the jokes?: (y/n) "))
if verification01 == "n":
firststage()
if verification01 == "y":
for i in range(jokeset_int):
joke = premiumjokemaker()
print(joke)
time.sleep(0.2)
verification001 = str(input("would you like to regenerate the jokes?: (y/n) "))
if verification001 == "y":
refirststage()
if verification001 == "n":
print("thank you for using the comedy machine.")
time.sleep(1)
os.system("cls")
print("the comedy machine will always return.")
time.sleep(1)
exit()
else:
print("please choose between y or n.")
The code for firststage().
# jokeset_func() receives input for the jokeset.
def jokeset_func():
jokeset = str is(input("how many jokes would you like to generate?: (1-10, codes allowed) "))
return jokeset
The code for jokeset_func(), used in the first part of firststage().
It’s quite simple. At first it globalizes jokesub1, jokeobj1, and jokeadj1 in order for the variables to work. Then it calls jokeset_func(), which asks for input on jokeset from 1-10. It then returns the value to firststage(). Back in firststage(), it does a little try block to test jokeset if it has a ValueError and converts it into a None, which means it’s neither a string or an integer, but it pretty much means a string here. Then it’s just code for error messages in case you put either a string or a number that isn’t between 1-10.
After that we get to the heart of firststage(). It is an elif statement when jokeset_int is not None and it is >= 1 or < 10. Then it prints some junk like “great” and asks for input on jokesub1 and jokeobj1, which are the subject and object variables, in case they weren’t obvious already. Once you’re done, it prints more “great” junk and requests a y/n input via verification01. If you input “n”, you get sent back to firststage(). If you input “y”, it prints a number of jokes depending on jokeset_int in a for loop.
"""
this is the jokemaker for if jokeset is from 1-9. it takes random jokes from the jokelist and
replaces the variables with the user's input.
"""
def jokemaker():
global jokesub1, jokeobj1
joketemp = random.choice(jokelist)
joke = joketemp.replace("{jokesub1}", jokesub1).replace("{jokeobj1}", jokeobj1)
return joke
This is the code for jokemaker(), the key function for generating jokes from the jokelist.
In the for loop, it calls jokemaker(), which selects a random joke from the jokelist through random.choice. Then it replaces {jokesub1} and {jokeobj1} (which are present in the joke templates as variable placeholders) with the variables themselves. It then returns everything to firststage().
In firststage(), after calling jokemaker(), it converts its’ value to joke. Then it loops print(“joke”) and time.sleep(0.2) (which delays the program) as many times as jokeset_int. Once it’s done, it again asks for a y/n input for joke regeneration through verification001. (creative, I know) If you type “n”, it prints some “thank you” stuff along with a cryptic “we will return” message. If you type “y”, it calls refirststage(), which as you guessed, is basically firststage(), but with some minor differences.
Remember what I said about 10 having an adjective as an option? Well, in the case that you put 10 into jokeset_int, it will basically do the same except it also asks you for jokeadj1 and chooses jokes from the premiumjokelist, which includes adjective variables in its jokes.
This project was on another level of frustration from my previous project. While being theoretically simple, this project was actually quite difficult as I had only learned basic Python beforehand. At first, I had planned to put 10 different levels for the comedy machine, hence the name firststage() for the function, but it soon got too tedious to code as I was struggling to make even the most basic mode work. The joke part was easy. Learning to use try blocks and f-strings in lists with an AI that went off the rails every 2 prompts was hard.
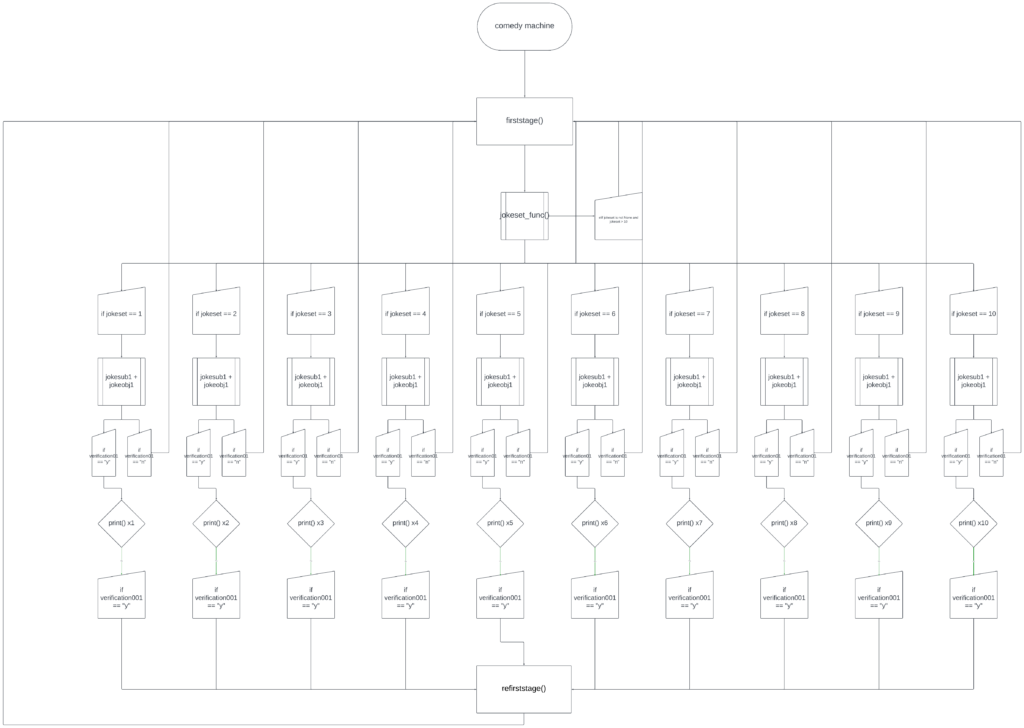
The original flowchart for the comedy machine. Note how instead of drawing jokes from a list, each number had its own unique jokes, which made it really boring.
As you can see from this crazy-looking flowchart, each number would have a unique set of jokes, as I hadn’t learnt how to use random.choice() or replace(). The AI had really helped me in places like those. I had also used the AI to generate the jokes present in the premiumjokelist, as I was tired of writing them.
I had learned a lot about Python in this project. From recursion to clearing the screen, I now feel a little more comfortable coding with it than before I started. I had also learned to control my frustration many, MANY times in this project. For example, early on in the project, I tried to implement codes into the input of jokeset_int. That didn’t work very well. It always gave me a ValueError, and the AI was being really dumb and giving me the wrong fixes. I had to do a crash course on variable types as well as try blocks to figure out how to fix it.
I feel like this project has a lot of potential and places to improve on. From a bigger jokelist to being able to save jokes or suggest new ones, the comedy machine can be something a lot more. However, for the time being, I think I’m gonna take a brain break and mess around with it for a little bit.
Aiden
One response to “The Story of How I Made a Comedy Machine in Pursuit of Endless Humor – Intro Post 2”
Outstanding project, Aiden. You’ve ticked all the boxes and clearly have a good understanding of basic Python.